ipyparallel
-Magic¶
[1]:
import ipyparallel as ipp
rc = ipp.Client()
[2]:
with rc[:].sync_imports():
import numpy
importing numpy on engine(s)
got unknown result: 05d7cec8-912387fc506b2f40c1471286
got unknown result: fa6fddcf-779a7f98c12df80a46c1e51f
[3]:
%px a = numpy.random.rand(2,2)
[4]:
%px numpy.linalg.eigvals(a)
Out[0:2]: array([0.65462266, 1.13932216])
Out[1:2]: array([ 0.83473139, -0.29322661])
Out[2:2]: array([0.05028953, 1.30003731])
Out[3:2]: array([0.99612347, 0.42574895])
[5]:
%px print("hi")
[stdout:0] hi
[stdout:1] hi
[stdout:2] hi
[stdout:3] hi
[6]:
%px %pylab inline
[stdout:0] Populating the interactive namespace from numpy and matplotlib
[stdout:1] Populating the interactive namespace from numpy and matplotlib
[stdout:2] Populating the interactive namespace from numpy and matplotlib
[stdout:3] Populating the interactive namespace from numpy and matplotlib
[7]:
%px plot(rand(100))
[output:0]
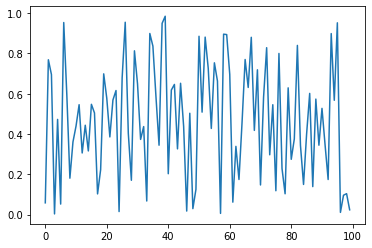
[output:1]
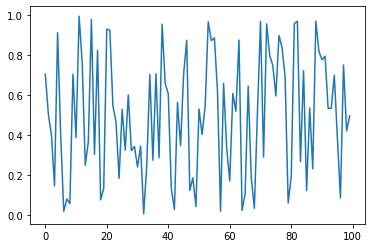
[output:2]
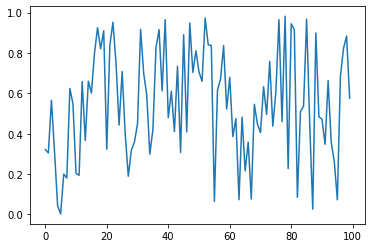
[output:3]
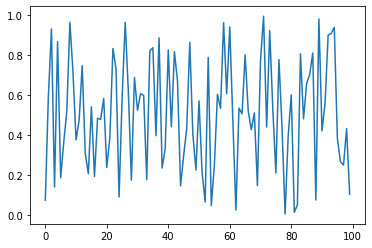
Out[0:5]: [<matplotlib.lines.Line2D at 0x1102e2b10>]
Out[1:5]: [<matplotlib.lines.Line2D at 0x10ea7f110>]
Out[2:5]: [<matplotlib.lines.Line2D at 0x113d70d50>]
Out[3:5]: [<matplotlib.lines.Line2D at 0x10fee7fd0>]
%%px
Cell Magic¶
--targets
, --block
und --noblock
[8]:
%%px --targets ::2
print("I am even")
[stdout:0] I am even
[stdout:2] I am even
[9]:
%%px --targets 1
print("I am number 1")
I am number 1
[10]:
%%px
print("still all by default")
[stdout:0] still all by default
[stdout:1] still all by default
[stdout:2] still all by default
[stdout:3] still all by default
[11]:
%%px --noblock
import time
time.sleep(1)
time.time()
[11]:
<AsyncResult: execute>
[12]:
%pxresult
Out[0:8]: 1570269717.543805
Out[1:8]: 1570269717.54598
Out[2:8]: 1570269717.5485692
Out[3:7]: 1570269717.548548
[13]:
%%px --block --group-outputs=engine
import numpy as np
A = np.random.random((2,2))
ev = numpy.linalg.eigvals(A)
print(ev)
ev.max()
[stdout:0] [-0.68780518 0.74951568]
[output:0]
Out[0:9]: 0.7495156792444352
[stdout:1] [0.75691299 1.08526474]
[output:1]
Out[1:9]: 1.0852647415435284
[stdout:2] [-0.2153414 1.46736661]
[output:2]
Out[2:9]: 1.467366609797983
[stdout:3] [0.55698336 0.13540831]
[output:3]
Out[3:8]: 0.5569833627025539
%pxresult
¶
[14]:
dview = rc[:]
[15]:
dview.block = False
%px print("hi")
%pxresult
[stdout:0] hi
[stdout:1] hi
[stdout:2] hi
[stdout:3] hi
[stdout:0] hi
[stdout:1] hi
[stdout:2] hi
[stdout:3] hi
%autopx
¶
[16]:
dview.block=True
[17]:
%autopx
%autopx enabled
[18]:
max_evals = []
for i in range(100):
a = numpy.random.rand(10, 10)
a = a + a.transpose()
evals = numpy.linalg.eigvals(a)
max_evals.append(evals[0].real)
[19]:
print("Average max eigenvalue is: %f" % (sum(max_evals)/len(max_evals)))
[stdout:0] Average max eigenvalue is: 10.209420
[stdout:1] Average max eigenvalue is: 10.214184
[stdout:2] Average max eigenvalue is: 10.071618
[stdout:3] Average max eigenvalue is: 10.132674
%pxconfig
¶
[3]:
%pxconfig --block
%px print("hi")
[stdout:0] hi
[stdout:1] hi
[stdout:2] hi
[stdout:3] hi
[4]:
%pxconfig --targets ::2
%px print("hi")
[stdout:0] hi
[stdout:2] hi
[5]:
%pxconfig --noblock
%px print("hi")
[5]:
<AsyncResult: execute>
[6]:
%pxresult
[stdout:0] hi
[stdout:2] hi
Mehrere aktive Views¶
Magics von ipyparallel
sind bestimmten DirectView
-Objekten zugeordnet. Der aktive View kann jedoch geändert werden mit dem Aufruf der activate()
-Methode auf einem View.
[3]:
even = rc[::2]
even.activate()
%px print("hi")
[3]:
<AsyncResult: execute>
[4]:
even.block = True
%px print("hi")
got unknown result: 98a3ad5c-00fa7f40e334c8a4186d5be5
[stdout:0] hi
[stdout:2] hi
Wenn ihr die Anischt aktiviert, könnt ihr auch einen Suffix angeben, so dass dieser bei einer ganzen Reihe von Magics zugeordnet werden kann ohne die bereits vorhandenen zu ersetzen.
[5]:
rc.activate()
[5]:
<DirectView all>
[6]:
even.activate("_even")
%px print("hi")
[stdout:0] hi
[stdout:1] hi
[stdout:2] hi
[stdout:3] hi
[7]:
%px_even print("We aren’t odd!")
[stdout:0] We aren’t odd!
[stdout:2] We aren’t odd!
Dieses Suffix wird am Ende aller Magics angewendet, also z.B.%autopx_even
, %pxresult_even
usw.
Der Einfachheit halber hat auch Client
eine activate()
-Methode, die einen DirectView
mit block = True
erstellt, aktiviert und die neue View zurückgibt.
Die anfänglichen Magics, die beim Erstellen eines Clients registriert werden, sind das Ergebnis des Aufrufs rc.activate()
mit Standardargumenten.
Engines als Kernel¶
Engines sind eigentlich dasselbe Objekt wie IPython-Kernels, mit der einzigen Ausnahme, dass Engines eine Verbindung zu einem Controller herstellen, während reguläre Kernels ihre Sockets direkt an Verbindungen zu ihrem Frontend binden.
Manchmal werdet ihr zum Debuggen oder Analysieren euer Frontend direkt mit einer Engine verbinden wollen, um eine direktere Interaktion zu ermöglichen. Auch dies könnt ihr tun, indem ihr die Engine anweist, ihren Kernel auch an euer Frontend bindet:
[ ]:
%px import ipyparallel as ipp; ipp.bind_kernel()
%px %qtconsole
Hinweis: Seid vorsichtig mit dieser Anweisung, da sie so viele QtConsole startet, wie Engines zur Verfügung stehen.
Alternativ könnet ihr euch die Verbindungsinformationen auch anzeigen lassen und so ermitteln, wie ihr eine Verbindung zu den Engines herstellen könnt, je nachdem, wo sie leben und wo Sie sich befinden:
[ ]:
%px %connect_info